TypeScript Tutorial for JavaScript Developers: Transitioning Made Easy
As a JavaScript developer, you’re likely familiar with the power and flexibility of the language, but what if there’s a way to make your JavaScript code more scalable, robust, and easier to maintain? Enter TypeScript, a superset of JavaScript that adds static typing to the language, making it an incredibly powerful tool for building large-scale applications.

If you’re already comfortable with JavaScript, transitioning to TypeScript can seem like a daunting task, but it doesn’t have to be. In this TypeScript tutorial for JavaScript developers, we’ll explore how to make the shift seamlessly, leveraging your existing knowledge while introducing you to the key concepts that make TypeScript a game-changer for modern web development.
What is TypeScript?
TypeScript is a statically typed, compiled superset of JavaScript. While JavaScript is dynamic (meaning types are determined at runtime), TypeScript adds type annotations that are checked at compile time. This helps catch errors early, provides better tooling (such as autocompletion and error checking), and makes the code more self-documenting, which is particularly helpful for larger, more complex codebases.
Since TypeScript compiles down to JavaScript, it’s fully compatible with any JavaScript code. You can start using TypeScript incrementally in your existing JavaScript projects, and it integrates well with modern frameworks like React, Angular, and Vue.
Why Should JavaScript Developers Learn TypeScript?
You might be asking, “Why should I learn TypeScript when I’m already proficient in JavaScript?” The answer lies in the benefits TypeScript brings to the table, especially for large-scale applications.
1. Better Developer Experience
One of the primary benefits of TypeScript is the improved developer experience. TypeScript's type system offers features like autocompletion, inline documentation, and error checking at compile time. These features can significantly reduce the likelihood of bugs, especially as your project grows in complexity. It also helps developers understand the code better since the types are explicitly defined.
2. Static Typing and Error Prevention
In JavaScript, types are determined at runtime, which means errors related to incorrect data types often don’t surface until the application is running. TypeScript’s static type system helps prevent these errors before they occur by enforcing type correctness at compile time. This can drastically reduce bugs and enhance the stability of your application.
3. Scalability
JavaScript is an excellent language for small projects, but as your applications grow in size, maintaining them can become challenging. TypeScript helps you manage and scale large codebases by introducing types, interfaces, and classes. These features allow for better organization of code, reducing the chances of errors and improving the maintainability of the project.
4. Compatibility with JavaScript
One of the biggest advantages of TypeScript is that it is a superset of JavaScript. This means that all valid JavaScript code is also valid TypeScript. You can gradually introduce TypeScript into an existing JavaScript project without the need for a complete rewrite, making it easier for JavaScript developers to transition.
Getting Started with TypeScript: A Smooth Transition
1. Set Up TypeScript in Your Project
To begin, you’ll need to install TypeScript in your project. The easiest way to do this is by using npm (Node Package Manager). Once you have Node.js and npm installed, you can install TypeScript globally by running the following command:
npm install -g typescript
You can also install it locally in your project:
npm install --save-dev typescript
After installation, you can initialize a tsconfig.json file, which contains the configuration for your TypeScript project. This file allows you to specify various compiler options and settings for your TypeScript code.
2. Start Writing TypeScript Code
Once you have TypeScript installed, you can start creating .ts files (TypeScript files). Since TypeScript is a superset of JavaScript, you can write JavaScript code in a .ts file without making any changes. However, the real power of TypeScript comes when you start adding type annotations to your variables and function parameters.
For example, you might write a simple function in TypeScript like this:
function greet(name: string): string {
return "Hello, " + name;
}
In this example, we explicitly defined the name parameter as a string and the function's return type as string. If you try to call the function with a non-string value, TypeScript will raise an error at compile time.
3. Leverage Type Inference
One of the most powerful features of TypeScript is type inference. Even if you don’t explicitly define types for your variables or functions, TypeScript will try to infer the correct type based on the value you assign.
For example:
let message = "Hello, TypeScript!";
TypeScript automatically infers that message is a string because it’s assigned a string value. This reduces the need for explicit type annotations while still providing type safety.
4. Understand TypeScript’s Key Features
As you transition from JavaScript to TypeScript, it’s essential to understand some key features of TypeScript that will make your development process more efficient:
- Interfaces: Interfaces allow you to define the shape of an object. This is useful for enforcing consistency in your codebase and ensuring that objects follow a specific structure.
- Enums: Enums are a powerful way to define a set of named constants. This is helpful when working with a limited set of values, such as status codes or user roles.
- Generics: Generics allow you to create reusable components that can work with any type. They provide flexibility while maintaining type safety.
- Decorators: TypeScript supports decorators, which are a special kind of declaration that can be attached to classes, methods, and properties to modify their behavior. This is particularly useful when working with frameworks like Angular.
5. Gradual Migration from JavaScript
If you’re working on an existing JavaScript project, you don’t need to convert everything to TypeScript all at once. You can migrate your project gradually, one file at a time. Start by renaming your .js files to .ts and fix any type errors that TypeScript highlights. Over time, you can progressively add type annotations and take full advantage of TypeScript’s features.
Key Tips for JavaScript Developers Transitioning to TypeScript
- Start with TypeScript Tutorial for Beginners If you’re just beginning with TypeScript, it's best to start with a TypeScript tutorial for beginners. These tutorials will walk you through the basics, like setting up your development environment and writing simple TypeScript code. By starting small, you can gain confidence and familiarity before diving into more advanced concepts.
- Use TypeScript’s Strict Mode TypeScript offers a strict mode that enables more rigorous type checking. By enabling strict mode in your tsconfig.json, you can ensure that TypeScript catches even the smallest issues in your code.
- Use Third-Party Type Definitions If you’re using third-party libraries or frameworks, you may need type definitions to take full advantage of TypeScript. Many popular libraries, such as React or Express, have TypeScript type definitions available through the @types namespace.
- Understand the Compiler and Tooling TypeScript provides robust tooling and integrations with popular editors like Visual Studio Code. Understanding the TypeScript compiler and how to configure it for your project will make your transition smoother.
Conclusion
If you're a JavaScript developer looking to learn TypeScript, the good news is that you already have a strong foundation. TypeScript is a powerful tool that can help you build more maintainable and scalable applications, and it’s easy to learn if you approach it step by step. By following this TypeScript tutorial and utilizing the features TypeScript offers, you can improve the quality of your code while continuing to build on your existing JavaScript expertise. Happy coding!
What's Your Reaction?
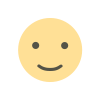
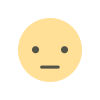
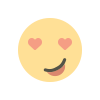

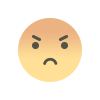

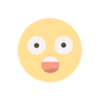